Merge pull request #1 from thatblindgeye/css-foundations
Add exercise instructions for css foundations
This commit is contained in:
commit
f7b0d534f6
|
@ -0,0 +1,21 @@
|
|||
# Methods for Adding CSS
|
||||
In this exerrcise, you're going to practice adding CSS to an HTML file using all three methods: external CSS, internal CSS, and inline CSS. You should only be using type selectors for this exercise when adding styles via the external and internal methods. You should also use keywords for colors (e.g. "blue" instead of using RGB or HEX values).
|
||||
|
||||
There are three elements for you to add styles to, each of which uses a different method of adding CSS to it, as noted in the outcome image below. All other exercises in this section will have a CSS file provided and linked for you, but for this exercise you will have to create the file and link it in the HTML file yourself. This is all about practicing using these different methods and getting the syntax right.
|
||||
|
||||
The properties you need to add to each element are:
|
||||
|
||||
* `div`: a red background, white text, a font size of 32px, center aligned, and bold
|
||||
* `p`: a green background, white text, and a font size of 18px
|
||||
* `button`: an orange background and a font size of 18px
|
||||
|
||||
## Desired Outcome
|
||||
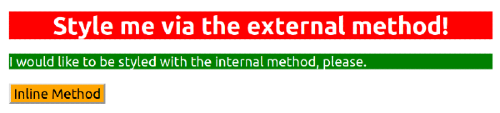
|
||||
|
||||
|
||||
### Self Check
|
||||
- Did you use all three methods of adding CSS to an HTML file?
|
||||
- Did you properly link the external CSS file in the HTML file?
|
||||
- Does the `div` element have CSS added via the external method?
|
||||
- Does the `p` element have CSS added via the internal method?
|
||||
- Does the `button` element have CSS added via the inline method?
|
|
@ -0,0 +1,14 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Methods for Adding CSS</title>
|
||||
</head>
|
||||
<body>
|
||||
<div>Style me via the external method!</div>
|
||||
<p>I would like to be styled with the internal method, please.</p>
|
||||
<button>Inline Method</button>
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,7 @@
|
|||
div {
|
||||
text-align: center;
|
||||
background-color: red;
|
||||
color: white;
|
||||
font-size: 32px;
|
||||
font-weight: bold;
|
||||
}
|
|
@ -0,0 +1,23 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Methods for Adding CSS</title>
|
||||
<link rel="stylesheet" href="solution.css">
|
||||
|
||||
<style>
|
||||
p {
|
||||
background-color: green;
|
||||
color: white;
|
||||
font-size: 18px;
|
||||
}
|
||||
</style>
|
||||
</head>
|
||||
<body>
|
||||
<div>Style me via the external method!</div>
|
||||
<p>I would like to be styled with the internal method, please.</p>
|
||||
<button style="background-color: orange; font-size: 18px;">Inline Method</button>
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,22 @@
|
|||
# Class and ID Selectors
|
||||
Knowing how to add class and ID attributes to HTML elements, as well as use their respective selectors, is invaluable, and it's important to practice using them.
|
||||
|
||||
There are several elements in the HTMl file provided, which you will have to add either class or ID attributes to as noted in the outcome image below. You will then have to add rules in the CSS file provided using the correct selector syntax. Look over the outcome image carefully, and try to keep in mind what elements look similarly styled (classes), which ones may be completely unique from the rest (ID), and which ones have slight variations from others (multiple classes).
|
||||
|
||||
It isn't entirely important what class or ID values you use, as the focus here is being able to add the attributes and use the correct selector syntax to style elements. For the colors in this exercise, try using a non-keyword value (RGB, HEX, or HSL). The properties you need to add to each element are:
|
||||
|
||||
* **All odd numbered elements**: a light red/pink background, and a list of fonts containing `Verdana` as the first option and `sans-serif` as a fallback
|
||||
* **The second element**: blue text and a font size of 36px
|
||||
* **The third element**: in addition to the styles for all odd numbered elements, add a font size of 24px
|
||||
* **The fourth element**: a light green background, a font size of 24px, and bold
|
||||
|
||||
Quick tip: in VS Code, you can change which format colors are displayed in (RGB, HEX, or HSL) by hovering over the color value in the CSS and clicking the top of the popup that appears!
|
||||
|
||||
## Desired Outcome
|
||||
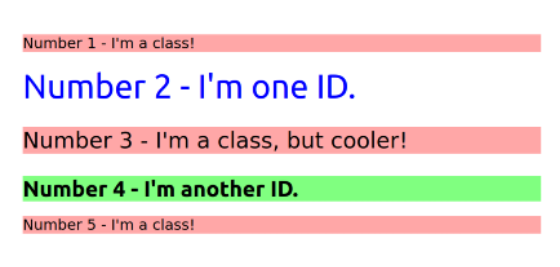
|
||||
|
||||
|
||||
### Self Check
|
||||
- Do the odd numbered `div` elements share a class?
|
||||
- Do the even numbered `div` elements have unique ID's?
|
||||
- Does the 3rd `div` element have multiple classes?
|
|
@ -0,0 +1,17 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Class and ID Selectors</title>
|
||||
<link rel="stylesheet" href="style.css">
|
||||
</head>
|
||||
<body>
|
||||
<p>Number 1 - I'm a class!</p>
|
||||
<div>Number 2 - I'm one ID.</div>
|
||||
<p>Number 3 - I'm a class, but cooler!</p>
|
||||
<div>Number 4 - I'm another ID.</div>
|
||||
<p>Number 5 - I'm a class!</p>
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,19 @@
|
|||
.odd {
|
||||
background-color: rgb(255, 167, 167);
|
||||
font-family: Verdana, sans-serif;
|
||||
}
|
||||
|
||||
.oddly-cool {
|
||||
font-size: 24px;
|
||||
}
|
||||
|
||||
#two {
|
||||
color: #0000ff;
|
||||
font-size: 36px;
|
||||
}
|
||||
|
||||
#four {
|
||||
background-color: hsl(120, 100%, 75%);
|
||||
font-size: 24px;
|
||||
font-weight: bold;
|
||||
}
|
|
@ -0,0 +1,17 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Class and ID Selectors</title>
|
||||
<link rel="stylesheet" href="solution.css">
|
||||
</head>
|
||||
<body>
|
||||
<p class="odd">Number 1 - I'm a class!</p>
|
||||
<div id="two">Number 2 - I'm one ID.</div>
|
||||
<p class="odd oddly-cool">Number 3 - I'm a class, but cooler!</p>
|
||||
<div id="four">Number 4 - I'm another ID.</div>
|
||||
<p class="odd">Number 5 - I'm a class!</p>
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,20 @@
|
|||
# Grouping Selectors
|
||||
|
||||
Let's build a little off the previous exercise, where you (hopefully) added multiple classes to a single element in order to apply two different rules to it.
|
||||
|
||||
Instead of having a single element with two different rules applied, though, you're instead going to give two elements each a unique class name, then add rules for styles that both elements share as well as their own unique styles. Make sure you take a good look at the outcome image below to see exactly what is unique about each element, and what both elements have in common.
|
||||
|
||||
This will help you further practice adding classes and using class selectors, so be sure you add the class attribute in the HTML file. For the remainder of these exercises, the format of any colors is entirely up to you, we trust you'll practice using the different values! The properties you need to add to each element are:
|
||||
|
||||
* **The first element**: a black background and white text
|
||||
* **The second element**: a yellow background
|
||||
* **Both elements**: a font size of 28px and a list of fonts containing `Helvetica` and `Times New Roman`, with `sans-serif` as a fallback
|
||||
|
||||
## Desired Outcome
|
||||
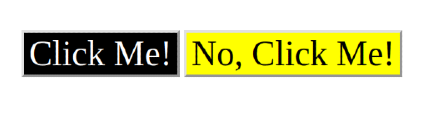
|
||||
|
||||
|
||||
### Self Check
|
||||
- Does each element have a unique class name?
|
||||
- Did you use the grouping selector for styles that both elements share?
|
||||
- Did you make separate rules for the styles unique to each element?
|
|
@ -0,0 +1,14 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Grouping Selectors</title>
|
||||
<link rel="stylesheet" href="style.css">
|
||||
</head>
|
||||
<body>
|
||||
<button>Click Me!</button>
|
||||
<button>No, Click Me!</button>
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,14 @@
|
|||
.inverted,
|
||||
.fancy {
|
||||
font-family: Helvetica, "Times New Roman", sans-serif;
|
||||
font-size: 28px;
|
||||
}
|
||||
|
||||
.inverted {
|
||||
background-color: black;
|
||||
color: white;
|
||||
}
|
||||
|
||||
.fancy {
|
||||
background-color: yellow;
|
||||
}
|
|
@ -0,0 +1,14 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Grouping Selectors</title>
|
||||
<link rel="stylesheet" href="solution.css">
|
||||
</head>
|
||||
<body>
|
||||
<button class="inverted">Click Me!</button>
|
||||
<button class="fancy">No, Click Me!</button>
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,21 @@
|
|||
# Chaining Selectors
|
||||
|
||||
Credits for the images in this exercise go to [Katho Mutodo](https://www.pexels.com/@photobykatho?utm_content=attributionCopyText&utm_medium=referral&utm_source=pexels) and [Andrea Piacquadio](https://www.pexels.com/@olly?utm_content=attributionCopyText&utm_medium=referral&utm_source=pexels).
|
||||
|
||||
With this exercise we've provided you a completed HTML file, so you will only have to edit the CSS file. It's more important to understanding how chaining different selectors works for this exercise than actually adding the attributes.
|
||||
|
||||
We have two images for you to style, each with two class names where one of the class names is shared. The goal here is to chain the selectors for both elements so that each have a unique style applied despite using a shared class selector. In other words, you want an element that has both X and Y to have one set of styles, while an element with X and Z has a completely different set of styles. We included the original images as well so that you can see how the styles you will be adding look in comparison, so do not add any styles to them.
|
||||
|
||||
The properties you need to add to each element are:
|
||||
|
||||
* Make the element with both the `avatar` and `proportioned` classes 300 pixels wide, then give it a height so that it retains its original square proportions (don't hardcode in a pixel value for the height!)
|
||||
* Make the element with both the `avatar` and `distorted` classes 200 pixels wide, then make its height twice as big as its width (here you should hardcode in a pixel value)
|
||||
|
||||
## Desired Outcome
|
||||
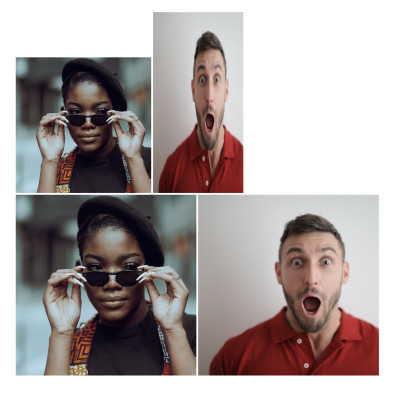
|
||||
|
||||
|
||||
### Self Check
|
||||
- Did you properly chain class selectors for each rule?
|
||||
- Does the `proportioned` image retain its original square proportions?
|
||||
- Does the `distorted` image end up looking squished and, well, distorted?
|
|
@ -0,0 +1,22 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Chaining Selectors</title>
|
||||
<link rel="stylesheet" href="style.css">
|
||||
</head>
|
||||
<body>
|
||||
<!-- Use the classes BELOW this line -->
|
||||
<div>
|
||||
<img class="avatar proportioned" src="./pexels-katho-mutodo-8434791.jpg" alt="">
|
||||
<img class="avatar distorted" src="./pexels-andrea-piacquadio-3777931.jpg" alt="">
|
||||
</div>
|
||||
<!-- Use the classes ABOVE this line -->
|
||||
<div>
|
||||
<img class="original-proportioned" src="./pexels-katho-mutodo-8434791.jpg" alt="">
|
||||
<img class="original-distorted" src="./pexels-andrea-piacquadio-3777931.jpg" alt="">
|
||||
</div>
|
||||
</body>
|
||||
</html>
|
Binary file not shown.
After Width: | Height: | Size: 20 KiB |
Binary file not shown.
After Width: | Height: | Size: 32 KiB |
|
@ -0,0 +1,9 @@
|
|||
.avatar.proportioned {
|
||||
height: auto;
|
||||
width: 300px;
|
||||
}
|
||||
|
||||
.avatar.distorted {
|
||||
height: 400px;
|
||||
width: 200px;
|
||||
}
|
|
@ -0,0 +1,20 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Chaining Selectors</title>
|
||||
<link rel="stylesheet" href="solution.css">
|
||||
</head>
|
||||
<body>
|
||||
<div>
|
||||
<img class="avatar proportioned" src="../pexels-katho-mutodo-8434791.jpg" alt="">
|
||||
<img class="avatar distorted" src="../pexels-andrea-piacquadio-3777931.jpg" alt="">
|
||||
</div>
|
||||
<div>
|
||||
<img class="original-proportioned" src="../pexels-katho-mutodo-8434791.jpg" alt="">
|
||||
<img class="original-distorted" src="../pexels-andrea-piacquadio-3777931.jpg" alt="">
|
||||
</div>
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,18 @@
|
|||
# Descendant Combinator
|
||||
Understanding how combinators work can become a lot easier when you start playing around with them and seeing what exactly is affected by them versus what isn't.
|
||||
|
||||
The goal of this exercise is to apply styles to elements that are descendants of another element, while leaving elements that *aren't* descendants of that element unstyled.
|
||||
|
||||
You can use either type or class selectors for this exercise, whichever you may feel you want to practice with more. The HTML file is setup (so no need to edit anything in it) where any combination of selectors will work, so if you're feeling adventurous you can even try combining a type *and* class selector for the descendant combinator.
|
||||
|
||||
The properties you need to add are:
|
||||
|
||||
* Only the `p` elements that are descendants of the `div` element should have a yellow background, red text, a font size of 20px, and center aligned
|
||||
|
||||
## Desired Outcome
|
||||
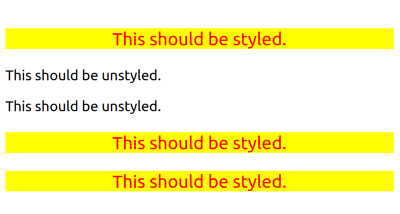
|
||||
|
||||
|
||||
### Self Check
|
||||
- Do the elements that contain the text "This should be styled" have the correct styles applied?
|
||||
- Do the elements that contain the text "This should be unstyled" have no styles applied?
|
|
@ -0,0 +1,21 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Descendant Combinator</title>
|
||||
<link rel="stylesheet" href="style.css">
|
||||
</head>
|
||||
<body>
|
||||
<div class="container">
|
||||
<p class="text">This should be styled.</p>
|
||||
</div>
|
||||
<p class="text">This should be unstyled.</p>
|
||||
<p class="text">This should be unstyled.</p>
|
||||
<div class="container">
|
||||
<p class="text">This should be styled.</p>
|
||||
<p class="text">This should be styled.</p>
|
||||
</div>
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,6 @@
|
|||
.container .text {
|
||||
background-color: yellow;
|
||||
color: red;
|
||||
font-size: 20px;
|
||||
text-align: center;
|
||||
}
|
|
@ -0,0 +1,21 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Descendant Combinator</title>
|
||||
<link rel="stylesheet" href="solution.css">
|
||||
</head>
|
||||
<body>
|
||||
<div class="container">
|
||||
<p class="text">This should be styled.</p>
|
||||
</div>
|
||||
<p class="text">This should be unstyled.</p>
|
||||
<p class="text">This should be unstyled.</p>
|
||||
<div class="container">
|
||||
<p class="text">This should be styled.</p>
|
||||
<p class="text">This should be styled.</p>
|
||||
</div>
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,15 @@
|
|||
# CSS Methods
|
||||
This final exercise for CSS Foundations is going to give you a closer look at the cascade, specificity and rule order in particular. Both the HTMl and CSS files are filled out for you, so instead of adding rules yourself you will simply be editing what is provided.
|
||||
|
||||
There are a few elements that have some sort of specificity or rule order issue in the provided CSS file. It's up to you to figure out what issue is affecting an element, and how to fix it. You can edit the CSS file by adding or removing selectors or moving stuff around, but you should not edit the HTML file or any of of the actual style declarations in the CSS.
|
||||
|
||||
There are multiple ways to solve this exercise, and we did our best to include all of the possible solutions for each element.
|
||||
|
||||
Issues with the cascade can be the bane for many when it comes to CSS, and while you won't be an expert from this exercise alone and there are other ways to deal with these issues, it is still super helpful to see how these issues affect our final styles and why its important to order rules carefully.
|
||||
|
||||
## Desired Outcome
|
||||
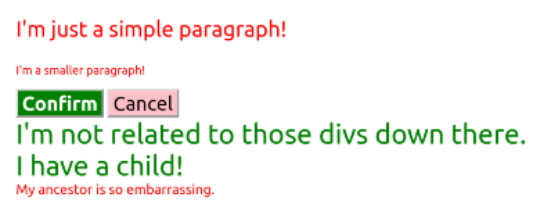
|
||||
|
||||
### Self Check
|
||||
- Did you make sure to not edit the HTML file?
|
||||
- If you added selectors to the CSS, do they target a valid HTML element?
|
|
@ -0,0 +1,22 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Fix the Cascade</title>
|
||||
<link rel="stylesheet" href="style.css">
|
||||
</head>
|
||||
<body>
|
||||
<p class="para">I'm just a simple paragraph!</p>
|
||||
<p class="para small-para">I'm a smaller paragraph!</p>
|
||||
|
||||
<button id="confirm-button" class="button confirm">Confirm</button>
|
||||
<button id="cancel-button" class="button cancel">Cancel</button>
|
||||
|
||||
<div class="text">I'm not related to those divs down there.</div>
|
||||
<div class="text">I have a child!
|
||||
<div class="text child">My ancestor is so embarrassing.</div>
|
||||
</div>
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,93 @@
|
|||
.para,
|
||||
.small-para {
|
||||
color: hsl(0, 100%, 50%);
|
||||
}
|
||||
|
||||
.para {
|
||||
font-size: 22px;
|
||||
}
|
||||
|
||||
.button {
|
||||
background-color: #ffc0cb;
|
||||
color: black;
|
||||
font-size: 20px;
|
||||
}
|
||||
|
||||
div.text {
|
||||
color: green;
|
||||
font-size: 28px;
|
||||
}
|
||||
|
||||
/* SOLUTION */
|
||||
|
||||
/*
|
||||
For the following rule, we removed it from its original position in the file:
|
||||
|
||||
.small-para {
|
||||
font-size: 12px;
|
||||
}
|
||||
|
||||
Then we placed it after the .para selector, taking advantage of the rule order since both selectors have the same specificity.
|
||||
|
||||
Another solution would be keeping it in its original place and just chaining selectors, giving this rule a higher specificity:
|
||||
|
||||
p.small-para {
|
||||
font-size: 12px;
|
||||
}
|
||||
*/
|
||||
|
||||
.small-para {
|
||||
font-size: 12px;
|
||||
}
|
||||
|
||||
/*
|
||||
For the following rule we removed the original .confirm selector:
|
||||
|
||||
.confirm {
|
||||
background: green;
|
||||
color: white;
|
||||
font-size: 20px;
|
||||
font-weight: bold;
|
||||
}
|
||||
|
||||
Then we used an ID selector instead, since it has a higher specificity than the .button selector.
|
||||
|
||||
Other solutions would be to simply move the .confirm rule below the .button rule, or to use the .button.confirm selector without moving it:
|
||||
|
||||
.button.confirm {
|
||||
background: green;
|
||||
color: white;
|
||||
font-size: 20px;
|
||||
font-weight: bold;
|
||||
}
|
||||
*/
|
||||
|
||||
#confirm-button {
|
||||
background: green;
|
||||
color: white;
|
||||
font-size: 20px;
|
||||
font-weight: bold;
|
||||
}
|
||||
|
||||
/*
|
||||
For the following rule we first removed it from its original position in the file:
|
||||
|
||||
.child {
|
||||
color: red;
|
||||
font-size: 14px;
|
||||
}
|
||||
|
||||
Then we added another selector to create a descendant combinator. If we only created a descendant combinator, the specificity would be tied with the div.text selector and it would come down to rule order, and if we only moved it the div.text selector would still have a higher specificity.
|
||||
|
||||
Another solution would be to keep the rule where it was and just replace the div selector with the .text selector:
|
||||
|
||||
.text .child {
|
||||
color: red;
|
||||
font-size: 14px;
|
||||
}
|
||||
*/
|
||||
|
||||
div .child {
|
||||
color: red;
|
||||
font-size: 14px;
|
||||
}
|
|
@ -0,0 +1,22 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
<title>Fix the Cascade</title>
|
||||
<link rel="stylesheet" href="solution.css">
|
||||
</head>
|
||||
<body>
|
||||
<p class="para">I'm just a simple paragraph!</p>
|
||||
<p class="para small-para">I'm a smaller paragraph!</p>
|
||||
|
||||
<button id="confirm-button" class="button confirm">Confirm</button>
|
||||
<button id="cancel-button" class="button cancel">Cancel</button>
|
||||
|
||||
<div class="text">I'm not related to those divs down there.</div>
|
||||
<div class="text">I have a child!
|
||||
<div class="text child">My ancestor is so embarrassing.</div>
|
||||
</div>
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,35 @@
|
|||
.para,
|
||||
.small-para {
|
||||
color: hsl(0, 100%, 50%);
|
||||
}
|
||||
|
||||
.small-para {
|
||||
font-size: 12px;
|
||||
}
|
||||
|
||||
.para {
|
||||
font-size: 22px;
|
||||
}
|
||||
|
||||
.confirm {
|
||||
background: green;
|
||||
color: white;
|
||||
font-size: 20px;
|
||||
font-weight: bold;
|
||||
}
|
||||
|
||||
.button {
|
||||
background-color: #ffc0cb;
|
||||
color: black;
|
||||
font-size: 20px;
|
||||
}
|
||||
|
||||
.child {
|
||||
color: red;
|
||||
font-size: 14px;
|
||||
}
|
||||
|
||||
div.text {
|
||||
color: green;
|
||||
font-size: 28px;
|
||||
}
|
Loading…
Reference in New Issue